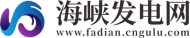
(相关资料图)
1 package com.damddos.waf.utils; 2 3 import com.jcraft.jsch.*; 4 import org.apache.commons.io.IOUtils; 5 6 import java.io.IOException; 7 import java.io.InputStream; 8 import java.nio.charset.Charset; 9 import java.util.Properties; 10 11 /** 12 * sftp工具类 13 * 14 * @author liYang 15 * @date 2023/04/10 16 */ 17 public class SftpUtil { 18 private static JSch jsch; 19 private static Session session = null; 20 private static Channel channel = null; 21 private static ChannelSftp channelSftp = null; 22 23 //服务器用户名 24 private String ftpUserName; 25 26 //服务器密码 27 private String ftpPassword; 28 29 //服务器ip 30 private String ftpHost; 31 32 //服务器端口 33 private String ftpPort; 34 35 public SftpUtil() { 36 } 37 38 public SftpUtil(String ftpUserName, String ftpPassword, String ftpHost, String ftpPort) { 39 this.ftpUserName = ftpUserName; 40 this.ftpPassword = ftpPassword; 41 this.ftpHost = ftpHost; 42 this.ftpPort = ftpPort; 43 } 44 45 /** 46 * 开启连接 47 */ 48 public ChannelSftp connect() { 49 jsch = new JSch(); 50 try { 51 // 根据用户名、主机ip、端口号获取一个Session对象 52 session = jsch.getSession(ftpUserName, ftpHost, Integer.valueOf(ftpPort)); 53 // 设置密码 54 session.setPassword(ftpPassword); 55 Properties config = new Properties(); 56 config.put("StrictHostKeyChecking", "no"); 57 // 为Session对象设置properties 58 session.setConfig(config); 59 // 设置连接超时为5秒 60 session.setTimeout(100 * 50); 61 // 通过Session建立连接 62 session.connect(); 63 // 打开SFTP通道 64 channel = session.openChannel("sftp"); 65 // 建立SFTP通道的连接 66 channel.connect(); 67 channelSftp = (ChannelSftp) channel; 68 } catch (JSchException e) { 69 e.printStackTrace(); 70 } 71 return channelSftp; 72 } 73 74 /** 75 * 关闭连接 76 */ 77 public void close() { 78 if (channel != null) { 79 channel.disconnect(); 80 } 81 if (session != null) { 82 session.disconnect(); 83 } 84 } 85 86 /** 87 * 判断文件夹路径是否存在 88 * 89 * @param directory 文件夹路径,如:/root/test/saveFile/ 90 */ 91 public boolean isDirExist(String directory) { 92 directory = null != directory && directory.endsWith("/") ? directory : directory + "/"; 93 boolean dirExist = false; 94 try { 95 SftpATTRS sftpATTRS = channelSftp.lstat(directory); 96 dirExist = sftpATTRS.isDir(); 97 } catch (Exception e) { 98 if (e.getMessage().equalsIgnoreCase("no such file")) { 99 dirExist = false;100 }101 }102 return dirExist;103 }104 105 /**106 * 创建一个文件夹(若整个路径都不存在会依次创建,若改路径已经存在则不会创建)107 *108 * @param createpath 要创建的文件夹路径,如:/root/test/saveFile/109 * @throws SftpException110 */111 public void createDir(String createpath) {112 createpath = null != createpath && createpath.endsWith("/") ? createpath : createpath + "/";113 if (!isDirExist(createpath)) {114 StringBuilder builder = new StringBuilder("/");115 String pathArry[] = createpath.split("/");116 for (String dir : pathArry) {117 if (!dir.equals("")) {118 builder.append(dir);119 builder.append("/");120 try {121 String path = builder.toString();122 if (!isDirExist(path)) {123 // 建立目录124 channelSftp.mkdir(path);125 }126 } catch (SftpException e) {127 e.printStackTrace();128 }129 }130 }131 }132 }133 134 /**135 * 删除文件136 *137 * @param deleteFile 要删除的文件路径,如:/root/test/saveFile/mylog.log138 */139 public void deleteFile(String deleteFile) {140 try {141 channelSftp.rm(deleteFile);142 } catch (Exception e) {143 e.printStackTrace();144 }145 }146 147 /**148 * 文件上传149 *150 * @param fileStram 文件输入流151 * @param upToPath 要上传到的文件夹路径152 * @param fileName 上传后的文件名153 */154 public void uploadFile(InputStream fileStram, String upToPath, String fileName) {155 upToPath = null != upToPath && upToPath.endsWith("/") ? upToPath : upToPath + "/";156 try {157 channelSftp.put(fileStram, upToPath + fileName);158 } catch (SftpException e) {159 e.printStackTrace();160 }161 }162 163 /**164 * 文件下载165 *166 * @param downlownPath 要下载的文件的所在文件夹路径167 * @param fileName 文件名168 * @return download 返回下载的文件流169 */170 public InputStream downloadFile(String downlownPath, String fileName) {171 downlownPath = null != downlownPath && downlownPath.endsWith("/") ? downlownPath : downlownPath + "/";172 InputStream download = null;173 try {174 download = channelSftp.get(downlownPath + fileName);175 } catch (SftpException e) {176 e.printStackTrace();177 }178 return download;179 }180 181 182 /**183 * 执行linux命令184 *185 * @param order 要执行的命令,(如,打印指定目录下的文件信息: ls -a /usr/local/kkFileView/kkFileView-4.0.0/bin/)186 * @return result 执行后返回的结果187 */188 public String excutOrder(String order) {189 String result = "";190 try {191 ChannelExec channelExec = (ChannelExec) session.openChannel("exec");192 channelExec.setCommand(order);193 channelExec.setErrStream(System.err);194 channelExec.connect();195 InputStream in = channelExec.getInputStream();196 result = IOUtils.toString(in, Charset.defaultCharset());197 } catch (JSchException e) {198 e.printStackTrace();199 } catch (IOException e) {200 e.printStackTrace();201 }202 return result;203 }204 205 }
项目需要。
关键词: